Whether you’re just starting out with Laravel or you’re looking to optimize an existing Laravel-based website, this article will provide you with a roadmap to supercharge your site’s performance and leave your competition in the dust.
At The Right Software, we pride ourselves on staying at the forefront of the latest web development trends and best practices. We’re confident that by implementing the strategies outlined in this article, you’ll be able to unlock the full potential of your Laravel website performance and provide your users with a lightning-fast experience.
Page Speed Insights Report
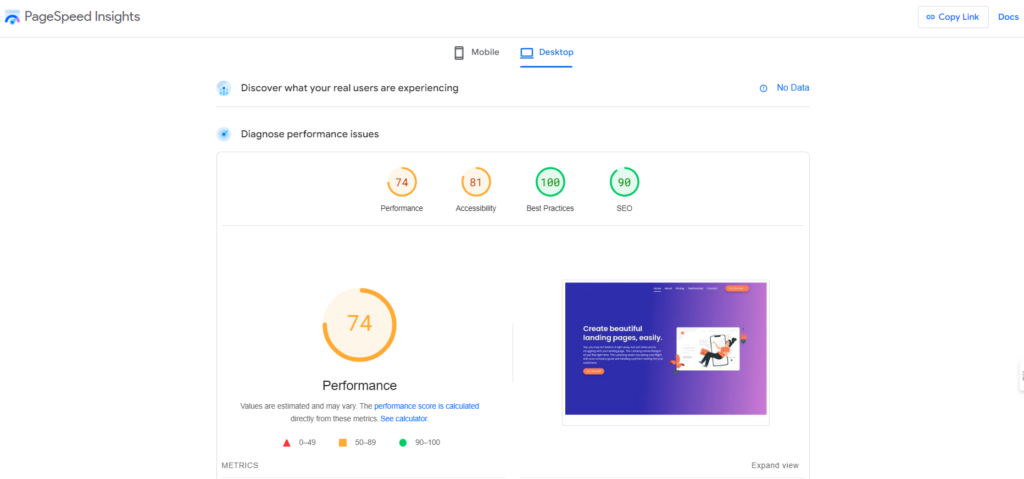
In addition to the optimization strategies outlined in this article, it’s important to monitor and optimize your website’s page speed. Page speed is a critical factor in both user experience and search engine optimization (SEO).
Google’s PageSpeed Insights is a valuable tool that can help you identify opportunities to improve your website’s performance. By addressing the recommendations provided by PageSpeed Insights, you can further enhance the speed and responsiveness of your Laravel-based website.
For example, after implementing the performance optimization steps detailed in this article, one of our recent client projects get a significant improvement in its PageSpeed Insights score. Before optimization, the website’s score was relatively low, but after applying these strategies, the score increased dramatically.
You can see the results in above image and you can check out your optimization efforts here: https://pagespeed.web.dev/
This is just one example of how the performance optimization techniques covered in this article can make a real difference in the speed and efficiency of your Laravel-powered website.
So, without further ado, let’s dive into the 15 proven steps to speed up your Laravel website performance:
1. Implement a Content Delivery Network (CDN)
A Content Delivery Network (CDN) is a network of servers distributed around the world that can serve your static assets (such as images, CSS, and JavaScript files) more efficiently than a single server.
By leveraging a CDN, you can reduce the latency experienced by your users by serving these assets from a server that is geographically closer to them. This can significantly improve the loading times of your website, especially for users located far from your primary server.
// Example of using the Laravel Horizon package to interact with a CDN
$image = Image::make('avatar.jpg')
->resize(100, 100)
->save('avatars/'.auth()->id().'.jpg');
Storage::disk('s3')->put('avatars/'.auth()->id().'.jpg', $image->stream());
// Example of using the Laravel Horizon package to interact with a CDN
$image = Image::make('avatar.jpg')
->resize(100, 100)
->save('avatars/'.auth()->id().'.jpg');
Storage::disk('s3')->put('avatars/'.auth()->id().'.jpg', $image->stream());
In this example, we’re using the Laravel Horizon package to store the resized avatar image on an Amazon S3 bucket, which can be served by a CDN like Amazon CloudFront.
By implementing a CDN, you can ensure that your website’s static assets are delivered to your users as quickly as possible, further enhancing the overall performance of your Laravel application.
2. Optimize Your Database Queries
One of the primary culprits behind slow-loading websites is inefficient database queries. Laravel’s Eloquent ORM makes it easy to interact with your database, but if you’re not careful, those queries can quickly become a performance bottleneck.
To optimize your database queries:
Use Eager Loading:
Eager loading allows you to load related models with a single query, reducing the number of database hits. Here’s an example:
// Without eager loading
$posts = Post::all();
foreach ($posts as $post) {
$post->user; // Triggers an additional query for each post
}
// With eager loading
$posts = Post::with('user')->get();
// Now the user data is loaded with the posts in a single query
// Without eager loading
$posts = Post::all();
foreach ($posts as $post) {
$post->user; // Triggers an additional query for each post
}
// With eager loading
$posts = Post::with('user')->get();
// Now the user data is loaded with the posts in a single query
Leverage Caching:
Implement caching strategies, such as Redis or Memcached, to store frequently accessed data and reduce database load. Here’s an example using Laravel’s built-in caching:
// Store data in cache
Cache::put('user_count', User::count(), now()->addHours(1));
// Retrieve data from cache
$userCount = Cache::get('user_count');
// Store data in cache
Cache::put('user_count', User::count(), now()->addHours(1));
// Retrieve data from cache
$userCount = Cache::get('user_count');
Profile Your Queries:
Use Laravel’s built-in profiling tools or third-party packages like Laravel Debugbar to identify and optimize slow queries.
Implement Pagination:
Instead of loading all records at once, use Laravel’s built-in pagination features to display content in manageable chunks.
$users = User::paginate(15);
// Display the users in a view
$users = User::paginate(15);
// Display the users in a view
3. Compress and Optimize Assets
Large, unoptimized assets like images, CSS, and JavaScript files can significantly slow down your website. Take the time to compress and optimize these assets to reduce file sizes and improve loading times.
Compress Images:
Use tools like TinyPNG or Imagemin to compress your images without sacrificing quality.
Minify CSS and JavaScript:
Use Laravel Mix or a tool like UglifyJS
to minify your CSS and JavaScript files, removing unnecessary whitespace and comments.
// Example of minifying JavaScript using UglifyJS
const UglifyJsPlugin = require('uglifyjs-webpack-plugin');
module.exports = {
plugins: [
new UglifyJsPlugin({
cache: true,
parallel: true,
sourceMap: true // set to true if you want JS source maps
})
]
};
// Example of minifying JavaScript using UglifyJS
const UglifyJsPlugin = require('uglifyjs-webpack-plugin');
module.exports = {
plugins: [
new UglifyJsPlugin({
cache: true,
parallel: true,
sourceMap: true // set to true if you want JS source maps
})
]
};
Enable Gzip Compression:
Configure your web server to enable Gzip compression for your HTML, CSS, and JavaScript files.
4. Optimize Caching Strategies
Caching is a powerful technique to improve website performance by reducing the amount of processing required for each request. Laravel provides several built-in caching mechanisms that you can leverage:
Related: 4 Best WordPress caching plugins compared (2019)
Page Caching:
Use Laravel’s built-in page caching to store the rendered output of your pages, reducing the need to re-render the entire page on each request.
Route::get('/', function () {
return Cache::remember('homepage', 60, function () {
return view('home');
});
});
Route::get('/', function () {
return Cache::remember('homepage', 60, function () {
return view('home');
});
});
View Caching:
Cache the compiled Blade views to avoid the overhead of compiling them on every request.
// In your view composer
View::composer('home', function ($view) {
$view->withData(Cache::remember('home_data', 60, function () {
return $this->fetchHomeData();
}));
});
// In your view composer
View::composer('home', function ($view) {
$view->withData(Cache::remember('home_data', 60, function () {
return $this->fetchHomeData();
}));
});
Route Caching:
Precompile your route definitions to improve routing performance.
php artisan route:cache
php artisan route:cache
Redis or Memcached:
Utilize high-performance caching solutions like Redis or Memcached to cache frequently accessed data.
// Example of caching with Redis
Redis::set('user:1:name', 'John Doe');
$name = Redis::get('user:1:name');
// Example of caching with Redis
Redis::set('user:1:name', 'John Doe');
$name = Redis::get('user:1:name');
5. Optimize Your Server Configuration
Use a Fast PHP Runtime:
Ensure you’re using a high-performance PHP runtime, such as PHP-FPM or HHVM, to handle your application’s PHP processing.Optimize Nginx/Apache Configuration:
Tune your web server’s configuration to optimize things like the number of worker processes, file caching, and more. Nginx Configuration for Performance – Discover tips for optimizing your web server configuration to improve performance.Use a Content Delivery Network (CDN):
Leverage a CDN to serve your static assets (images, CSS, JavaScript) from a network of servers closer to your users, reducing latency.6. Implement Asynchronous Processing
For long-running or resource-intensive tasks, such as sending emails or processing background jobs, consider using asynchronous processing to offload the work and keep your website responsive.
Laravel provides a robust queue system that allows you to dispatch jobs to various queue drivers, such as Redis, Amazon SQS, or even a database. By moving these tasks to a background queue, you can ensure your website’s main request-response cycle remains fast and efficient.
// Example of dispatching a job to the queue
dispatch(new SendEmailJob($user, $email));
// Example of dispatching a job to the queue
dispatch(new SendEmailJob($user, $email));
7. Utilize Caching Middleware
Leveraging Laravel’s built-in caching middleware can significantly improve the performance of frequently accessed pages. By caching the response of your routes, you can reduce the amount of processing required for each request, leading to faster load times for your users.
To implement this optimization, you can add the cache.headers
middleware to your route definitions in the RouteServiceProvider
. This middleware will cache the response of your routes for a specified duration, reducing the need to re-render the content on subsequent requests.
// In your RouteServiceProvider
protected function mapWebRoutes()
{
Route::middleware('web', 'cache.headers:public;max-age=3600;etag')
->namespace($this->namespace)
->group(base_path('routes/web.php'));
}
// In your RouteServiceProvider
protected function mapWebRoutes()
{
Route::middleware('web', 'cache.headers:public;max-age=3600;etag')
->namespace($this->namespace)
->group(base_path('routes/web.php'));
}
By using this middleware, you can take advantage of Laravel’s built-in caching capabilities to improve the overall performance of your website.
8. Implement Lazy Loading
Lazy loading is a technique that can significantly reduce the initial page load time by loading resources (such as images, iframes, and other content) only when they are needed, rather than loading everything upfront.
You can implement lazy loading in your Laravel application by using a JavaScript library like Lazysizes
. Lazysizes provides a simple and efficient way to lazy load images, iframes, and other content on your pages.
<!-- Example of using the Lazysizes library for lazy loading -->
<img class="lazyload" data-src="image.jpg" alt="My Image">
<!-- Example of using the Lazysizes library for lazy loading -->
<img class="lazyload" data-src="image.jpg" alt="My Image">
By utilizing lazy loading, you can ensure that your users only load the content they need, which can lead to faster initial load times and a more responsive website experience.
9. Implement Lazy Loading for Images
In addition to lazy loading for general resources, you can also specifically optimize the loading of images on your website. This can be achieved by using the native loading="lazy"
attribute or by leveraging a library like Lazysizes.
<!-- Example of using the native lazy loading attribute -->
<img src="image.jpg" loading="lazy" alt="My Image">
<!-- Example of using the native lazy loading attribute -->
<img src="image.jpg" loading="lazy" alt="My Image">
The loading="lazy"
attribute instructs the browser to defer the loading of the image until it is near the viewport, reducing the initial page load time and improving the perceived performance of your website.
Alternatively, you can use a library like Lazysizes, which provides a more robust and customizable lazy loading solution for images.
By implementing lazy loading for images, you can ensure that only the necessary images are loaded, leading to faster load times and a more efficient user experience.
10. Preload Critical Assets
Preloading critical assets, such as CSS, fonts, and JavaScript files, can help ensure that these resources are prioritized and loaded as soon as possible, improving the perceived performance of your website.
You can use the <link rel="preload">
HTML tag to preload critical assets. This tells the browser to fetch and cache these resources before they are needed, allowing them to be served more quickly when the page is loaded.
<!-- Example of preloading a critical CSS file --">
<link rel="preload" href="/css/critical.css" as="style" onload="this.onload=null;this.rel='stylesheet'">
<!-- Example of preloading a critical CSS file --">
<link rel="preload" href="/css/critical.css" as="style" onload="this.onload=null;this.rel='stylesheet'">
By preloading your critical assets, you can ensure that your website’s most important resources are available to the user as soon as possible, creating a faster and more responsive experience.
11. Utilize Service Workers
Service workers are a powerful feature of modern web browsers that allow you to cache and serve static assets from the browser’s cache, reducing the need to fetch these resources from the server on repeat visits.
Implementing service workers in your Laravel application can significantly improve the performance and offline capabilities of your website. Service workers can cache HTML, CSS, JavaScript, and other static assets, allowing your website to load much faster for returning users.
// Example of a basic service worker implementation
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/sw.js')
.then(registration => {
console.log('Service Worker registered');
})
.catch(error => {
console.log('Service Worker registration failed:', error);
});
});
}
// Example of a basic service worker implementation
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/sw.js')
.then(registration => {
console.log('Service Worker registered');
})
.catch(error => {
console.log('Service Worker registration failed:', error);
});
});
}
By leveraging service workers, you can create a more reliable and responsive user experience, even in areas with poor internet connectivity.
12. Optimize Third-Party Scripts
Third-party scripts, such as analytics trackers, advertising platforms, and social media widgets, can have a significant impact on your website’s performance if not properly optimized.
To ensure that third-party scripts don’t slow down your website, consider the following strategies:
Defer Script Loading:
Use the defer
attribute on your <script>
tags to instruct the browser to download the script in parallel but execute it only after the HTML parsing is complete.
<!-- Example of deferring a third-party script -->
<script defer src="https://example.com/script.js">
<!-- Example of deferring a third-party script -->
<script defer src="https://example.com/script.js"></script>
Lazy Load Third-Party Scripts:
Only load third-party scripts when they are needed, such as when a user interacts with a specific widget or feature.
Minimize Third-Party Script Usage:
Carefully evaluate the necessity of each third-party script and remove any that are not essential to your website’s functionality.
By optimizing the loading and usage of third-party scripts, you can ensure that they don’t negatively impact the performance of your Laravel website.
13. Utilize Webpack for Asset Bundling
Webpack is a powerful tool that can help you optimize the bundling and loading of your JavaScript and CSS assets. By using Webpack, you can reduce the number of HTTP requests required to load your website, leading to improved performance.
// Example Webpack configuration
module.exports = {
entry: './resources/js/app.js',
output: {
filename: 'js/app.js',
},
plugins: [
new MiniCssExtractPlugin({
filename: 'css/app.css',
}),
],
};
// Example Webpack configuration
module.exports = {
entry: './resources/js/app.js',
output: {
filename: 'js/app.js',
},
plugins: [
new MiniCssExtractPlugin({
filename: 'css/app.css',
}),
],
};
Webpack allows you to bundle your assets, minify them, and apply other optimizations, such as code splitting and tree shaking. By enhancing Webpack’s capabilities, you can ensure that your website’s assets are delivered to the user in the most efficient way possible.
14. Leverage HTTP/2 and Server Push
HTTP/2 is the latest version of the HTTP protocol, and it introduces several performance-enhancing features, such as multiplexing, header compression, and server push.
You can leverage HTTP/2 and server push in your Laravel application to deliver critical assets to the client more efficiently. Server push allows the server to proactively push resources to the client, reducing the number of round trips required to load a page.
# Example Nginx configuration for HTTP/2 and server push
server {
listen 443 ssl http2;
# SSL configuration...
location / {
# ...
http2_push /css/critical.css;
http2_push /js/app.js;
}
}
# Example Nginx configuration for HTTP/2 and server push
server {
listen 443 ssl http2;
# SSL configuration...
location / {
# ...
http2_push /css/critical.css;
http2_push /js/app.js;
}
}
By implementing HTTP/2 and server push, you can optimize the delivery of your website’s assets, leading to faster load times and a more responsive user experience.
15. Optimize Queries with Scopes and Accessors
In addition to the database optimization techniques mentioned earlier, you can further optimize your queries by leveraging Eloquent scopes
and accessors
.
Eloquent scopes allow you to encapsulate complex query logic into reusable methods, making your code more readable and maintainable. Accessors, on the other hand, can help you transform and format data before it is returned to your application.
// Example of an Eloquent scope
class User extends Model
{
public function scopeActive($query)
{
return $query->where('is_active', true);
}
}
// Example of an Eloquent accessor
class Post extends Model
{
public function getExcerptAttribute()
{
return Str::limit($this->content, 200);
}
}
// Example of an Eloquent scope
class User extends Model
{
public function scopeActive($query)
{
return $query->where('is_active', true);
}
}
// Example of an Eloquent accessor
class Post extends Model
{
public function getExcerptAttribute()
{
return Str::limit($this->content, 200);
}
}
By optimizing your queries with scopes and accessors, you can reduce the amount of data returned from the database, leading to faster response times and improved overall performance.
If you want to optimize your Codeigniter website then you must read out our 8 Steps to CodeIgniter performance optimization
Conclusion
So this is all we did for Laravel performance optimization. It is not necessary that all of the above listed steps must be applied in order to optimize the speed of a web page. But depending on a particular case, you will need to use a combination of these steps.
The 15 proven steps we’ve covered in this article range from optimizing your database queries and compressing assets to leveraging caching strategies, implementing asynchronous processing, and utilizing a Content Delivery Network (CDN). By selectively applying the most relevant strategies for your website, you can achieve significant performance improvements and deliver a superior user experience.
Remember, website optimization is an ongoing process, and you may need to revisit and fine-tune these strategies over time as your website evolves. If you’re unsure about how to implement these steps or need further assistance, our team of Laravel experts at The Right Software is here to help.
Contact us today to learn more about our Laravel development services and how we can help you take your website to the next level. Together, we’ll supercharge your Laravel website performance and leave your competition in the dust.