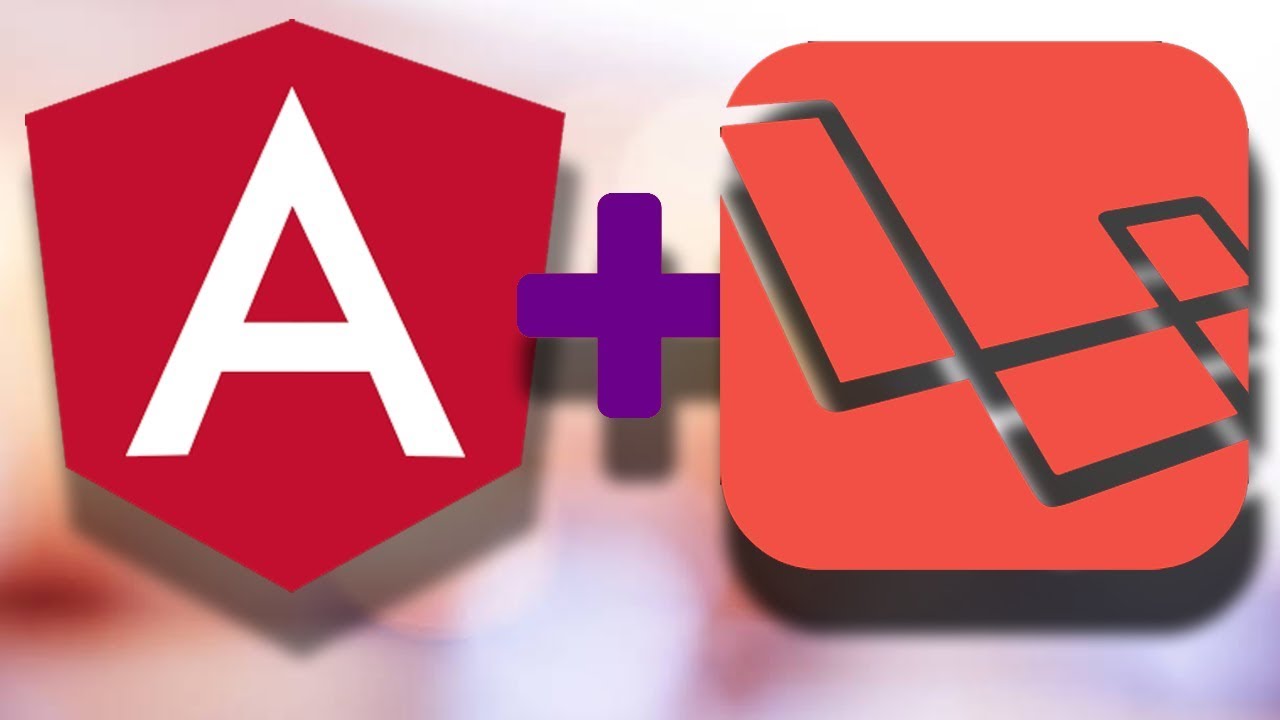
At The Right Software, we commonly use PHP Laravel 5 for database integration and Angular for frontend single page applications. So it makes sense to write about integrating PHP Laravel APIs with Angular frontend.
1. Develop Laravel API
Check out how to develop RESTful Laravel APIs. Here we’ll do a quick job as its not the scope of the tutorial. Lets setup the database first. We follow proper Laravel structure through commands and code.1.1 Create Laravel API migration
For PHP Laravel APIs to integrate in Angular 7, we have to write APIs in api.php file in Laravel which is in the routes folder in Laravel project structure. For the sake of this article, we are using example of User API. For User API to fetch and save data, you have to first setup database table. For that you have to write the migration for the table the command to create migration is written below.php artisan make:migration create_user_tableAfter creating a migration, you have to make a model for the table that you have created. Which can be made by command written below.
1.2 Laravel model command
php artisan make:model UserOr short hand for creating migration and model both you will use this command
php artisan make:model User -mAfter using this command the migration and model is made.
1.3 Laravel migration code example
Now you can write table in the migration. Below is the example to write table in migration.public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->increments('id');
$table->string('email')->unique();
$table->string('first_name');
$table->string('last_name');
$table->string('password');
$table->enum('account_status',['active','pending','rejected'])->default('pending');
$table->enum('gender',['male','female']);
$table->timestamps();
});
}
You can see the more about how to write table in the migration go to this link:
https://laravel.com/docs/5.7/migrations. After writing the table you have to run this command to migrate the table into the database
php artisan migrateAfter migration, you have to write a method into the model that was created to get, update, or add data into the table. For an API to run you have to make,
Route::post('allUsers','UserController@getAllUsers');
1.4 Laravel controller command
php artisan make:controller UserController
1.5 Laravel controller code example
The controller function will look like this.public function getAllUsers(){
$count = User::All()->count();
if($count > 0){
$users = User::role(['user','admin','dealer'])->get();
return response()->json($users);
}else{
return response()->json("Users Not Found");
}
}
You can also make resource and collection to return the response. You can go this link and check the eloquent resources and collection https://laravel.com/docs/5.7/eloquent-resources.
So in this api the api is using UserController Method getllAllUsers to get the users from the database. After that you have to serve the laravel project to use these apis into angular project.
2. Integrate Laravel API in Angular
First setup your angular project using this command. Using Angular Cli.ng new project_name
After that make your first component. For making component use this command.
ng generate component Users
// Or short hand:
ng g c Users
Four files will be generated after running that command. After that design your page in the html file
and in the ts file your code goes there. After that you have to make service to integrate the api calls that has been written in the Laravel so to create a service run this command.
ng generate service User
// Or short hand:
ng g s User
After running this command 2 file will be generated you will write the code in the ts file name users.service.ts there you will first import the HttpClient first. Then go to the environment folder and write the the api served url of the laravel project for local the url will look like this.
http://127.0.0.1:8000
The Laravel project will always serve on the 8000 port.
After importing the HttpClient then import the environment.ts file in the users service and get the api url from there. For importing just import the HttpClient into the constructor of the service.ts file.
After importing our service constructor will look like this.
apiUrl: any;
constructor(private http: HttpClinet){
this.apiUrl = environment.apiUrl;
}
After that write a function to to call the API of the Laravel and we will use the HttpClient in the function an example is written below.
getAllUsers() {
return this.http.get(this.apiUrl + 'allUsers/')
.pipe(map((response: any) => {
return response.json();
}));
}
This function is returning the data of all the users and parse then into the JSON response and return from where this function will get called.
After creating this function to get the data from the Laravel API now we will use this function into the the component that we have created first. To use the users service function import the users.service.ts file into the constructor of the users component. So this is the example to use the this service into the component.
The constructor will look like this after importing
constructor(private usersService: UsersService){
}
Here you can change the variable name for the user Service. You can set the variable name as you like. There is an ngOnInit() function in every component this is the first function to get called when ever the project gets served or this component is called. To get all the users from the service function that is calling Laravel API call to get all users the code will look like this.
allusers: any;
getUsers(){
this.usersService. getAllUsers()
.subscribe({
response => {
this.allusers=response;
},
});
}
Here we have made first a global variable that is storing the response from the call into itself so that the response can be used the users.component.html file and anywhere in the users.component.ts file.
The getUsers function is calling the service function gellAllUsers to get the user and save it into the local variable. Now you can use this variable in whatever the way you like into your html page to show it on the webpage.
In Angular, you can also make the model to map the response also so for that you need to make a file names users.model.ts there is no specific command for that. So the model is made for the easiness to use the response of the API. Example is given below.
export class User{
id: number;
first_name: string;
last_name: string;
email: string;
gender: string;
status: string;
}
For mapping the response on to the model we will use Observables. Observable provide the support for passing the messages between creators of the observables and the subscribers in our application. Here the subscriber will be our getUsers function in the users.component.ts file and the Observable will be defined in the users.service.ts.
For using observers we will import the rxjs Observable into the users.service.ts file. Then after that the Observable will be used like this as shown in example below.
getUsers(): Observable<{data: Users[]}>{
const headers = new HttpHeaders({'Content-Type': 'application/json'});
return this.http.get<{status_code: number, data: Users[], message: string}>(this.apiUrl +
'allUsers', {headers: headers});
}
Here the observable is parsing the response into the user model which we have created above as we are getting all the users from the API response so we are use Users[] array. And to get its parsed over here you have to format the response also in the Laravel API function. After this we also need to change the users.component.ts file so it will look like this.
allusers: Users[];
First we need to make your allUsers variable type to Users[] as the model and as we have done it in the service file the the gellUsers function will able to map response now.
getUsers(){
this.usersService. getAllUsers()
.subscribe({
response => {
this.allusers=response;
},
});
}
Now it has made it easy for you to get the variable easily that are in the users model if you want to see the firstname of all the users you can easily do this
this.allusers.foreach(element =>{
console.log('First Name',element.firstname);
})
as firstname is the variable that is in the model of Users.
If you follow this detailed tutorial, you can learn to write the APIs in Laravel and to integrate them in Angular project. If you get stuck, send us an email below, we’ll be right with you. If you are looking to Develop a web based application in Angular with Laravel API, you can hire us with confidence.