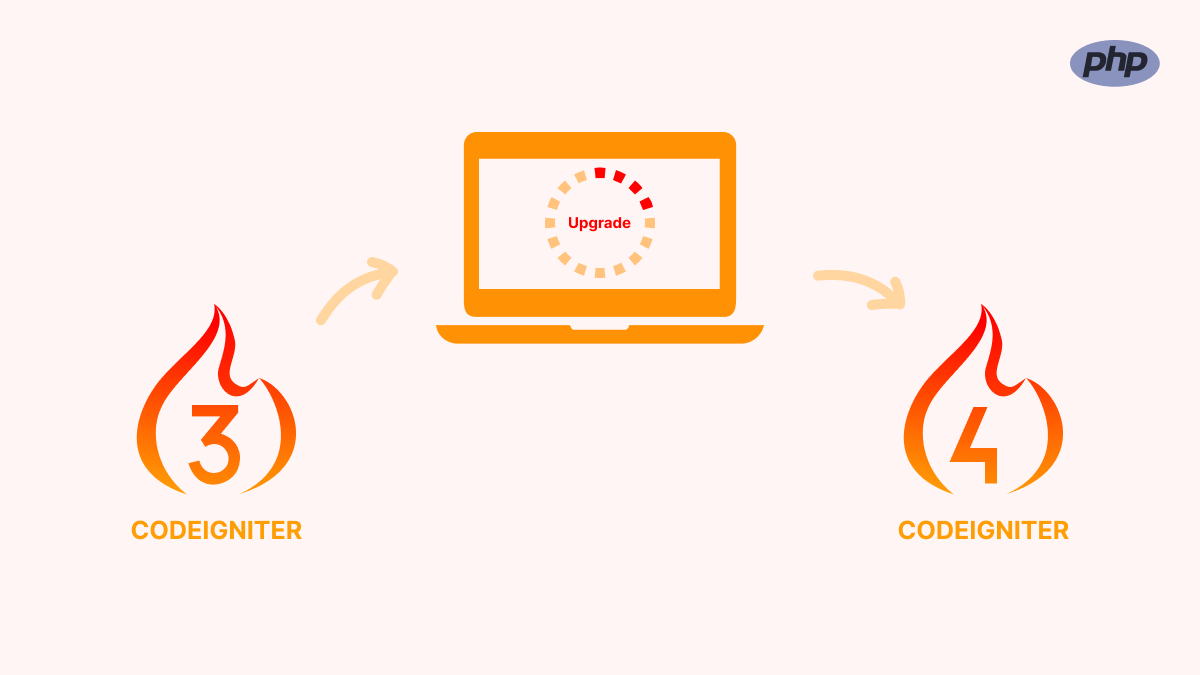
CodeIgniter 4 embraces contemporary PHP practices, incorporating functionalities like PSR-4 autoloading and namespaces. These features are instrumental in averting naming conflicts, fostering scalability, and ensuring a more sustainable codebase. Additionally, with Composer support, it streamlines package management.
Version 4 introduces a command-line interface, empowering developers to execute diverse tasks like database migrations without relying on a web browser. This CLI capability significantly augments automation, streamlining routine development processes.
We’ve previously covered a related topic in our article at UPGRADING PHP CODEIGNITER 2.X VERSION PROJECT TO 3.X . This resource provides in-depth insights into the process of upgrading PHP CodeIgniter projects from version 2.x to 3.x, offering valuable information and guidance for a more informed and successful development journey.
Let’s explore a sustainable codebase with Composer support and a powerful command-line interface for streamlined development.
Server requirements for CodeIgniter 4
PHP and Required Extensions
- int
- mbstring
- json
Optional PHP Extensions
The following PHP extension should be enabled on your server:
mysqlnd
(if you use MySQL)
curl
(if you use CURLRequest)
imagick
(if you use Image class ImageMagickHandler)
gd
(if you use Image class GDHandler)
simplexml
(if you format XML)
The following PHP extensions are required when you use a Cache server:
memcache
(if you use Cache class MemcachedHandler with Memcache)
redis
(if you use Cache class RedisHandler)
The following PHP extensions are required when you use PHPUnit:
dom
(if you use TestResponse class)
libxml
(if you use TestResponse class)
xdebug
(if you use CIUnitTestCase::assertHeaderEmitted()
)
Supported Databases
A database is required for most web application programming. Currently supported databases for CodeIgniter 4 are:
- MySQL via the MySQLi driver (version 5.1 and above only)
- PostgreSQL via the Postgre driver (version 7.4 and above only)
- SQLite3 via the SQLite3 driver
- Microsoft SQL Server via the SQLSRV driver (version 2005 and above only)
- Oracle Database via the OCI8 driver (version 12.1 and above only)
CodeIgniter 4 Installation
There are two ways to install CodeIgniter 4:
- Composer Installation
- Manual Installation
CI4 installation via Composer
CodeIgniter 4 requires composer 2.0.14 or later, if you were using the Git repository to store your code then the vendor folder would normally be in “git ignored”. In such instances, when cloning the repository onto a new system, executing a ‘composer update‘ becomes necessary.
Composer create-project codeigniter4/appstarter new-project
The command above will create a new-project folder
CodeIgniter 4 manual installation:
Download the latest version and extract it to become your project’s root
Initial CI 4 configuration
Open the app/config/App.php
file
- $baseURL: Set your baseURL, you may use
.env
to handle your environment variables - $indexPage: If you do not want to include index.php in your site URLs, set $indexPage to ‘’. The setting will be used when the framework generates your site URLs.
You might have to adjust your web server settings to enable access to your site using a URL that does not include ‘index.php’.
Remove the index.php file
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^(.*)$ index.php/$1 [L]
When you use an nginx server you can specify a location block and use the try_files directive to achieve the same effect as we did with the Apache configuration above.
server {
listen 80;
listen [::]:80;
server_name example.com;
root /var/www/example.com/public;
index index.php index.html index.htm;
location / {
try_files $uri $uri/ /index.php$is_args$args;
}
location ~ \.php$ {
include snippets/fastcgi-php.conf;
# With php-fpm:
fastcgi_pass unix:/run/php/php8.1-fpm.sock;
# With php-cgi:
# fastcgi_pass 127.0.0.1:9000;
}
error_page 404 /index.php;
# deny access to hidden files such as .htaccess
location ~ /\. {
deny all;
}
}
This configuration enables URLs without “index.php” in them and uses CodeIgniter’s “404 – File Not Found” for URLs ending in “.php“.
It will first search for a file or directory matching the URI (creating the full path to each file from the settings of the root and alias directives) and then send the request to the index.php file with any arguments. Configure Database
app/Config/Database.php
file with a text editor and set your database settings. Alternatively, these can be set in your .env
file. Namespaces
CodeIgniter 4 is built for PHP 7.4+, and everything in the framework is namespace, except for the helper and lang files.
Application Structure
- The application folder has been renamed to app and the framework now has system folders, with the same explanation as earlier.
- The framework now provides a public folder, intended as the document root for your app.
- defined (‘BASEPATH’) or exit(‘direct script access not allowed’); The line is not essential because files outside the public folder are not accessible in the standard setting. In addition, CI4 no longer defines a constant BASEPATH, so remove the line in all files.
- This is also a writable folder, so keep some data, logs, and session data.
- The app folder looks very similar to the application for CI3, with some name changes and some subfolders moved to a writable folder.
- There is no nested application/core folder anymore, as we have a different mechanism for extending framework components.
Routing
- Auto-routing is disabled by default. You need to set all routes as default.
- If you want to use auto-routing like CodeIgniter 3, you need to enable auto-routing.
- CI4 also has optional new more secure auto-routing.
app/Config/Routes.php
file. In it, you will see that it creates an instance of the RouteCollection class ($routes)
which allows you to define your own routing criteria.
When you specify a route, you select a method corresponding to the HTTP verb (request method) like.
<?php
$routes->get('/', 'Home::index');<br ?--> $routes->post('/form', 'Home::formsubmit');
$routes->put('/update', 'Home::updateform');
$routes->patch('/updatefield', 'Home::updateformfield');
?>
Model-View-Controller Architecture
CodeIgniter is uses MVC design pattern. Thus, the changes in the Model, View, and Controller are most important.
- Models: Models in CodeIgniter 4 are classes that handle database operations, encapsulating queries, data operation and validation.
- View: Views in CodeIgniter 4 are responsible for displaying the user interface using HTML templates, which can include dynamic data passed from controllers.
- Controller: Controllers receive requests, process data using models, and pass the data to views for rendering. They handle user interactions and decide which models and views to utilize for a specific request.
Upgrade Models
- First, move all model files to the folder
app/Models;
or you use this php sparkmake:model
userModel command to generate one by one model then you just add methods inside this model. - Add this line on top of the page after php open tag
namespace App\Models;
- After that you add this line
use Codeigniter\Models;
- Replace CI_Model with extends Model
- Instead of CI3’s
$this->load->model(‘x’) now use $this->x = new x();
following namespaced conventions of your component. Alternatively, you can use themodel() function $this->x = model(‘x’);
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserContact extends Model
{
}
?>
Upgrade Views
- First move all views to the folder
app/Views
- Change this syntax from CodeIgniter 3,
from
$this->load->view(‘directory_name/file_name’);
to returnview(‘directoryname/filename’);
fromecho $this->load->view(‘file_name’);
toecho view(‘fileName’);
- Remove all defined(‘BASEPATH’) OR exit(‘No direct script access allowed’);
<html>
<head>
<title>><?= esc($title)</title>
</head>
<body>
<h1><?= esc($heading) ?></h1>
<h3>My Todo List</h3>
</body>
</html>
Upgrade Controllers
- First move all controller files to the folder
app/Controllers
or run the commandphp spark make:controller
userController then move app method inside this file - Add this line after opening php tag
namespace App\Controllers;
after that adduse App\Controllers\BaseController;
- Remove the line defined(‘BASEPATH’) OR exit(‘No direct script access allowed’);
<?php
namespace App\Controllers;
use App\Controllers\BaseController;
class Helloworld extends BaseController
{
public function index($name)
{
return 'Hello ' . esc($name) . '!';
}
}
?>
Class loading
- CodeIgniter 4 no longer supports “Superobject”, in which references to framework components are magically injected as properties of your controller.
- Classes are instantiated where necessary, and framework components are managed by services.
- Autoloader automatically handles PSR-4 style class locating within the app (app folder) and CodeIgniter (i.e system folder) top-level namespaces. Composer with Autoloading support.
- You can configure classloading to support whatever application structure you’re most comfortable with, including the “HMVC” style.
- CodeIgniter 4 provides factories that can load classes and share an instance like
$this->load
in CI3.
Upgrading Libraries
- Your app classes can still go inside
app/Libraries
, but they do not have to. - Instead of CI3’s
$this->load->library('x');
you can now use$this->x = new \App\Libraries\X();
, Otherwise, you can useFactories: $this->x = \CodeIgniter\Config\Factories::libraries('x');
. - Some libraries from CI 3 no longer exists in CI 4.
- CodeIgniter 3 input corresponds to CodeIgniter 4 IncomingRequest.
- CodeIgniter 3 output corresponds to CodeIgniter 4 Responses.
Some other libraries, which exist in both CI versions, can be upgraded with some changes
Upgrade Helpers
- Helpers in CodeIgniter 4 remain similar to previous versions, although some have undergone simplification.
- Starting from version 4.3.0, you can autoload helpers using
'app/Config/Autoload.php'
similar to the approach in CI3. - However, certain helpers from CodeIgniter 3 no longer exist in Version 4. Notably, the CAPTCHA Helper, Email Helper, Path Helper, and Smiley Helper have been removed. Functions from these helpers must be implemented using alternative methods.
- Additionally, the Download Helper from CodeIgniter 3 has been eliminated, requiring the use of the Response object instead of the deprecated ‘
force_download()
‘ function. Refer to the ‘Force File Download‘ documentation for details. - While the Language Helper from CI3 has been removed, the ‘lang()’ function remains available and functional in CI4. Refer to ‘lang()’ for further information.
- The Typography Helper in CI3 is now the Typography Library in CI4.
- The Directory Helper and File Helper from CI3 have been merged into the Filesystem Helper in CI4.
- Functions from the String Helper in CI3 are now included within the Text Helper in CI4.
- In CodeIgniter 4, ‘
redirect()
‘ operates differently from CI3. Instead of directly redirecting and ending script execution, ‘redirect()
‘ in CodeIgniter 4 returns a RedirectResponse instance. Therefore, it needs to be explicitly returned. - To adapt from CI3’s ‘
redirect('login/form')
‘, you should replace it with ‘return redirect()->to('login/form')
‘ in CodeIgniter 4 for proper functionality.
Upgrade Hooks
- In CodeIgniter 4, Hooks have been replaced by Events.
- Instead of the CodeIgniter 3 method like
$hook['post_controller_constructor']
, you now utilize Events as follows:Events::on('post_controller_constructor', ['MyClass', 'MyFunction']);
, ensuring to include the namespaceCodeIgniter\Events\Events;
. - Events are universally enabled and accessible across the application.
- Previously available hook points such as pre_controller and post_controller have been phased out in favor of Controller Filters.
- Similarly, hook points like display_override and cache_override have been removed due to the removal of base methods associated with them.
- The post_system hook point has been relocated to occur just before the final rendered page is sent.
Extending the Framework
- In CodeIgniter 4, there’s no requirement for a specific ‘core‘ folder to house custom framework component extensions or replacements such as ‘MY_…‘ classes.
- Unlike earlier versions, you’re not confined to placing MY_X classes within the libraries folder to extend or replace CI4 components. You have the flexibility to position these classes wherever you prefer.
- To load your custom components instead of the default ones, create these classes in your preferred location and add the corresponding service methods in ‘
app/Config/Services.php
.’ - Refer to the ‘Creating Core System Classes’ documentation for detailed instructions.
<?php
namespace App\Models;
use CodeIgniter\Model;
class UserContact extends Model
{
}
?>
Conclusion: Upgrade CodeIgniter 4 or Hire a CI4 Expert
This article is a detailed roadmap for developers upgrading from CodeIgniter 3 to CodeIgniter 4. It focuses on fundamental changes, stressing the need to check if the server meets the new PHP requirements first.
Exploring the updated folder structure is a core aspect, explaining how namespaces help organize controllers, models, and views in the ‘app’ directory.
It talks about using the HMVC pattern in CodeIgniter 4, which makes development more organized and scalable.
The article also covers steps to update code syntax and libraries for compatibility with the new version.
Readers are guided through configuring routes, highlighting differences from the older version. It provides tips for updating existing code, like adjusting namespaces and configuration files, ensuring a smooth transition to CodeIgniter 4.
Besides tech tips, it stresses the importance of backup, version control, and thorough testing during the migration. Additionally, it shares valuable resources such as official documentation, forums, and tools for a successful upgrade.
Related: Best Practices of Codeigniter API Development
And if you want to optimize performance of your CodeIgniter website: 8 Steps to CodeIgniter performance optimization
Thank you for joining us on this exploration of Upgrading PHP CodeIgniter 3.x version to 4.x version. We hope this article has provided you with valuable insights and knowledge to enhance your web development projects. You can contact The Right Software to hire expert CodeIgniter 4 developer to perform these steps for your website.