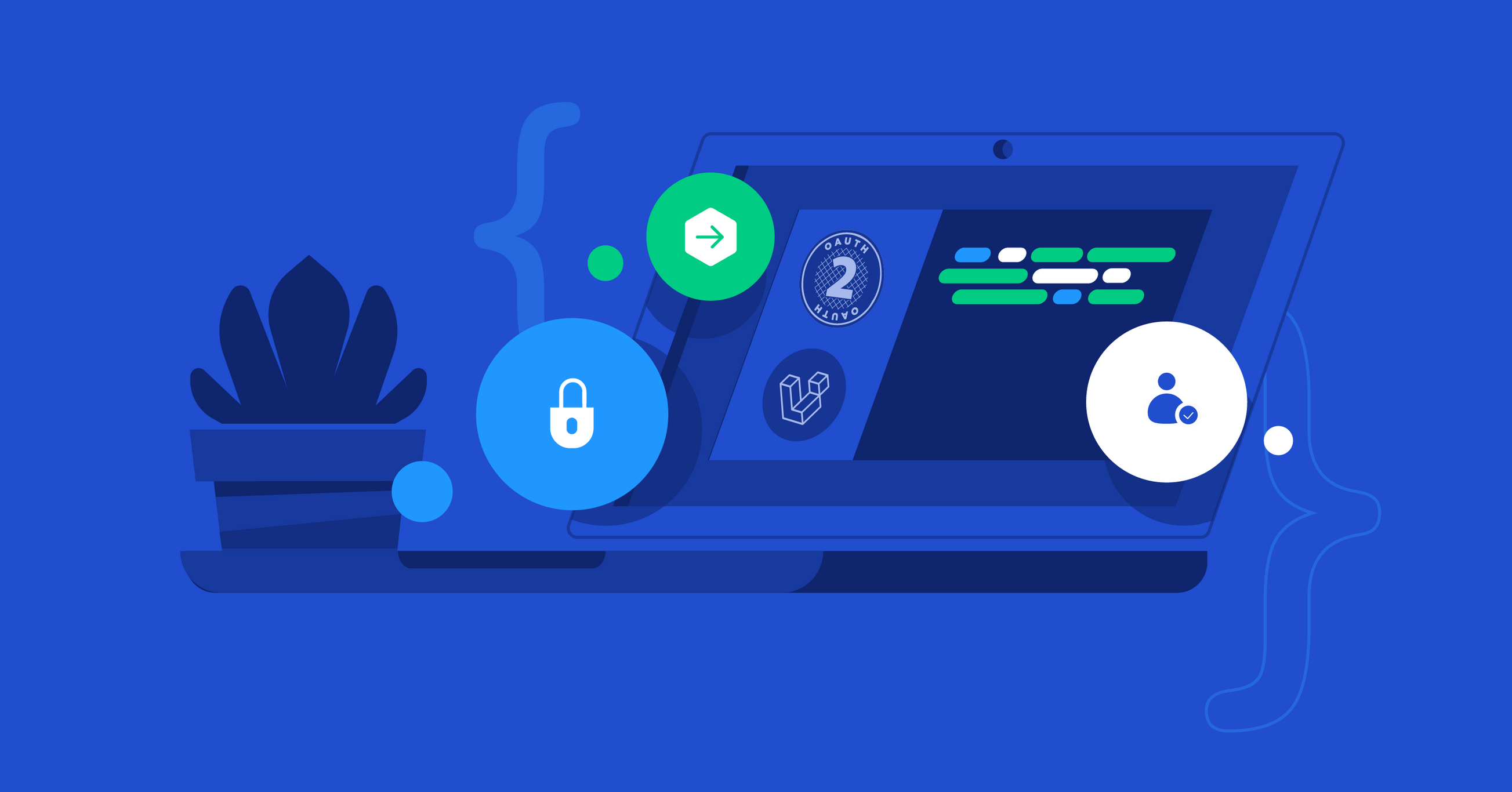
Laravel is one of the most popular and widely used PHP frameworks. Laravel saves a lot of time and cost of development by easing common tasks used in the majority of web applications, such as authentication, routing, sessions, caching, and more.
Laravel has the flexibility to extend its power with plugins and packages, thanks to fast-growing community of developers, we have tons of packages that provide specific functionalities and can be freely reused anywhere in the application.
What are Laravel packages?
Packages, also known as plugins, are pieces of code that can be integrated into existing software to extend its functionalities. Most web applications have common functionalities like authentication, Roles, Permissions, rather than coding them in all the time again we create packages that take care of these repetitive tasks and use them in any application, it is also known as DRY (Don’t Repeat Yourself) method
How to install packages in Laravel?
To install a package, we need a package manager also called dependency manager, Laravel uses composer to install and manage packages and their versions in Laravel.
Laravel has a file named composer.json
where all the packages and their versions are listed. Composer makes it really easy to install any package; just add a line (package name and version) in the composer.json file or use composer require
command.
composer require packageowner/packagename To use the installed package we initialize it: $package = new Package;”
Best Laravel Packages
Socialite
Most of the users like to have quicker to way get into an application without fillings heavy forms, that’s the reason social login has become a must-have feature for web applications. Socialite offers a simple and easy way to handle OAuth authentication. It allows the users to log in via some of the most popular social networks and services including Facebook, Twitter, Google, GitHub, and BitBucket.
To install socialite in your Laravel application run this command in the root directory
“composer require laravel/socialite” Open the file config/app.php and add following in the providers array “Laravel\Socialite\SocialiteServiceProvider::class,” In the aliases array of the same file, add ‘Socialite’ => Laravel\Socialite\Facades\Socialite::class,
Now the socialite is installed but in order to use it we need to add credentials for the OAuth providers.
Add the following to config/services.php
'facebook' => [ 'client_id' => env('FACEBOOK_CLIENT_ID'), 'client_secret' => env('FACEBOOK_CLIENT_SECRET'), 'redirect' => env('FACEBOOK_URL'), ], 'twitter' => [ 'client_id' => env('TWITTER_CLIENT_ID'), 'client_secret' => env('TWITTER_CLIENT_SECRET'), 'redirect' => env('TWITTER_URL'), ], 'google' => [ 'client_id' => env('GOOGLE_CLIENT_ID'), 'client_secret' => env('GOOGLE_CLIENT_SECRET'), 'redirect' => env('GOOGLE_URL'), ],
Add the following to .env file with your Oauth credentials
FACEBOOK_CLIENT_ID= FACEBOOK_CLIENT_SECRET= FACEBOOK_URL=http://localhost:8000/login/facebook/callback TWITTER_CLIENT_ID= TWITTER_CLIENT_SECRET= TWITTER_URL=http://127.0.0.1:8000/login/twitter/callback GOOGLE_CLIENT_ID= GOOGLE_CLIENT_SECRET= GOOGLE_URL=http://localhost:8000/login/google/callback
Roles and permissions
Most of the time we need an admin panel to manage our application, and we have different types of users for the application. Same application is used by different types of users that can have different privileges, to restrict access to unprivileged areas of the application we need to create roles and permissions.
Rather than creating this functionality all over again in every application, we have different packages to manage it. Spatie’s Laravel-Permission Package provides us with these functionalities and is very easy to use. It takes care of creating roles, assigning permissions to roles, assigning permissions to specific users, and much more.
You can install the package via composer:
“composer require spatie/laravel-permission” Open the file config/app.php and add following in the providers array 'providers' => [ // ... Spatie\Permission\PermissionServiceProvider::class, ];
It creates migrations for managing roles and permissions in DB and also creates a config file config/permissions, run the following command to publish these files.
php artisan vendor:publish –provider=”Spatie\Permission\PermissionServiceProvider”
Run the migrations: After the config and migration have been published and configured, you can create the tables for this package by running:
“php artisan migrate”
Intervention Image
Intervention Image is a PHP image handling and manipulation library providing an easier and more expressive way to create, edit, and compose images. The package includes ServiceProviders and Facades for easy Laravel integration.
Run the following command to install this package
“composer require intervention/image”
Open the config/app.php file and update the following code in the file.
$providers => [ ......, 'Intervention\Image\ImageServiceProvider' ], $aliases => [ ......, 'Image' => 'Intervention\Image\Facades\Image' ] Example: // usage inside a laravel route Route::get('/', function() { $img = Image::make('foo.jpg')->resize(300, 200); return $img->response('jpg'); });
Laravel Meta Manager
By using Laravel Meta Manager, you can optimize your website’s SEO, thereby helping your website rank higher on the first page of the search engine.
SEO Features
- Standard Meta Tags
- Facebook OpenGraph Meta Tags
- Twitter Card Meta Tags
- Dublin Core Meta Tags
- Link Tags
Run the following to install this package with Composer
“composer require davmixcool/laravel-meta-manager”
Update your config/app.php file with following code
'providers' => [ Davmixcool\MetaManager\MetaServiceProvider::class, ];
Then run following command to publish the config of Laravel Meta Manager.
php artisan vendor:publish --provider="Davmixcool\MetaManager\MetaServiceProvider"
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
@include('meta::manager', [
'title' => 'My Example Title',
'description' => 'This is my example description',
'image' => '',
])
</head>
Laravel Backup
This Laravel package creates a backup of all your files within an application. It creates a zip file that contains all files in the directories you specify along with a dump of your database. You can store a backup on any file system.
Run the following to install this package with Composer
composer require backup-manager/Laravel
Then, you’ll need to select the appropriate packages for the adapters that you want to use.
s3 or google cs composer require league/flysystem-aws-s3-v3 Dropbox composer require srmklive/flysystem-dropbox-v2 Rackspace composer require league/flysystem-rackspace Sftp composer require league/flysystem-sftp GCS composer require superbalist/flysystem-google-storage Scheduling Backups It's possible to schedule backups using Laravel's scheduler. protected function schedule(Schedule $schedule) { $environment = config('app.env'); $schedule->command( "db:backup --database=mysql --destination=s3 --destinationPath=/{$environment}/projectname --timestamp="Y_m_d_H_i_s" --compression=gzip" )->twiceDaily(13,21); }
Ziggy – Use your Laravel routes in JavaScript
Ziggy provides a JavaScript route()
helper function that works like Laravel’s, making it easy to use your Laravel named routes in JavaScript.
Run the following to install this package with Composer
“composer require tightenco/Ziggy” Example Laravel routes/web.php Route::get('posts', fn (Request $request) => /* ... */)->name('posts.index'); app.js route('posts.index'); With parameters Laravel routes/web.php Route::get('posts/{post}', fn (Request $request, Post $post) => /* ... */)->name('posts.show'); app.js route('posts.show', 1); // 'https://ziggy.test/posts/1' route('posts.show', [1]); // 'https://ziggy.test/posts/1' route('posts.show', { post: 1 }); // 'https://ziggy.test/posts/1'
Laravel Activity Log
For the audit and security most of the applications log data. Laravel logs the activities and save them in laravel.log file. There is a package by spatie that provides the functionality to log data and save it to DB and retrieve to show in dashboard.
The spatie/laravel-activitylog
package provides easy to use functions to log the activities of the users of your app. It can also automatically log model events. The Package stores all activity in the activity_log
table.
Run the following to install this package with Composer
“composer require spatie/laravel-activitylog”
Publish migrations with following command
php artisan vendor:publish --provider="Spatie\Activitylog\ActivitylogServiceProvider" --tag="activitylog-migrations"
Example
You can log and activity like following
activity()->log('Look, I logged something');
You can retrieve all activity using the Spatie\Activitylog\Models\Activity model.
Activity::all();
Laravel Cashier
Laravel is an outstanding platform for creating membership and ecommerce application, the most important part of these applications is a payment method. To receive payment for purchases or recurring membership fees, we can use laravel cashier that provides an expressive, fluent interface to Stripe’s subscription billing services
It handles almost all of the heavy lifting for subscription billing code you are writing. In addition to basic subscription management, Cashier can handle coupons, swapping subscription, subscription “quantities”, cancellation grace periods, and even generate invoice PDFs.
Run the following to install this package with Composer
“composer require laravel/cashier”
Cashier creates its own migrations to save billing data in DB, use following command to migrate.
“php artisan migrate”
If you need to overwrite the migrations that ship with Cashier, you can publish them using the vendor:publish Artisan command:
php artisan vendor:publish --tag="cashier-migrations".
To use cashier we need to add “Billable” trait to our billing model.
use Laravel\Cashier\Billable; class User extends Authenticatable { use Billable; }
Next we need API key and secret from stripe add these to you .env file
STRIPE_KEY=your-stripe-key STRIPE_SECRET=your-stripe-secret
Laravel Debugbar
Laravel Debugbar is one of the essential packages laravel developers who are looking for a better way of debugging their apps without wasting time at looking at error logs files or using the function like dd() and ddd() for inspecting data.
This is a package to integrate PHP Debug Bar with Laravel. It includes a ServiceProvider to register the debugbar and attach it to the output. You can publish assets and configure it through Laravel. It bootstraps some Collectors to work with Laravel and implements a couple custom DataCollectors, specific for Laravel. It is configured to display Redirects and Ajax Requests.
Run the following to install this package with Composer
composer require barryvdh/laravel-debugbar –dev
Add provider and aliase in app/config.php
'providers' => [ .... Barryvdh\Debugbar\ServiceProvider::class, ], 'aliases' => [ .... 'Debugbar' => Barryvdh\Debugbar\Facade::class, ]
To enable the debugger make APP_DEBUG=True in .env file
Debugbar::info($object); Debugbar::error('Error!'); Debugbar::warning('Watch out…'); Debugbar::addMessage('Another message', 'mylabel');

Laravel adjacency list
This Laravel Eloquent extension provides recursive relationships using common table expressions (CTE).
A Common Table Expression, also called as CTE in short form, is a temporary named result set that you can reference within a SELECT, INSERT, UPDATE, or DELETE statement.
Run the following to install this package with Composer
composer require staudenmeir/laravel-adjacency-list:"^1.0"
Following are some of the relationships provided by the package:
- ancestors(): The model’s recursive parents.
- ancestorsAndSelf(): The model’s recursive parents and itself.
- bloodline(): The model’s ancestors, descendants and itself.
- children(): The model’s direct children.
- childrenAndSelf(): The model’s direct children and itself.
- descendants(): The model’s recursive children.
- descendantsAndSelf(): The model’s recursive children and itself.
- parent(): The model’s direct parent.
- parentAndSelf(): The model’s direct parent and itself.
- rootAncestor(): The model’s topmost parent.
- siblings(): The parent’s other children.
- siblingsAndSelf(): All the parent’s children.
Example Usage
$ancestors = User::find($id)->ancestors; $users = User::with('descendants')->get(); $users = User::whereHas('siblings', function ($query) { $query->where('name', '=', 'John'); })->get(); $total = User::find($id)->descendants()->count(); User::find($id)->descendants()->update(['active' => false]); User::find($id)->siblings()->delete();
Important note before using any package
Although packages are very useful and time-saving, they shouldn’t be used blindly, always run tests and check reviews before using any package.
Always check if a package has opened up a potential security loophole before publishing or deploying your application. Avoid using unnecessary packages, if a package is no longer remove all its dependency.
Conclusion: use these packages frequently
Our list of packages ends here, but, of course, there are lot more out there. You can find a list of available packages at Packalyst. Currently, there are more than 15000 packages listed.
If you are looking to develop web applications using Laravel, you can always discuss with us. We provide expert and mature website solutions for our clients.