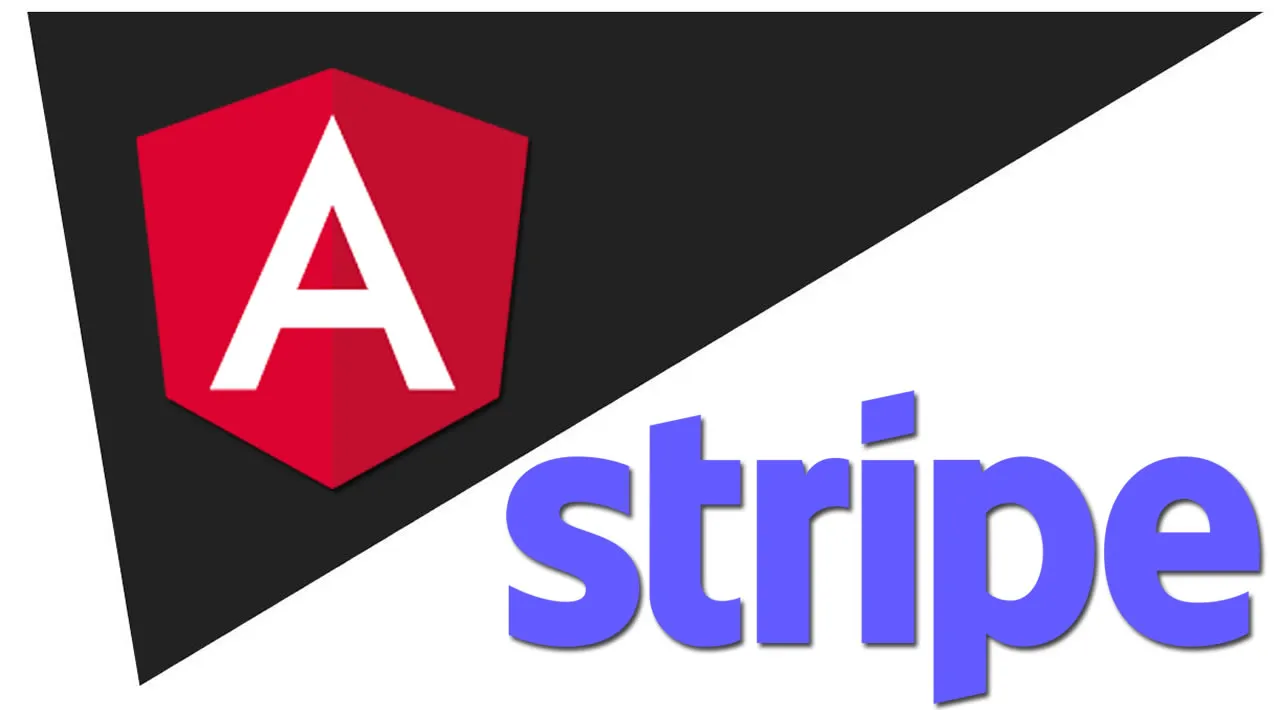
Understanding Stripe Angular Integration
Stripe is a widely adopted payment gateway that offers a simple and secure way to integrate financial services into your software. Angular, on the other hand, is a powerful JavaScript framework for building modern, single-page client applications. The integration allows you to explore the best of both worlds – the flexibility and robustness of Angular combined with the fast and reliable payment processing capabilities of Stripe.
The following stripe angular integration example will work with the latest angular version at the time of publishing this article. This article will help the developer with stripe angular integration because it is one of the most used methods in payment forms.
Prerequisites for stripe angular integration
Before we dive into the integration process, ensure that you have the following set up on your development machine:
- Node.js and NPM: Make sure you have the latest stable version of Node.js and NPM installed. You can download them from the official Node.js website (https://nodejs.org).
- Angular CLI: Install the Angular CLI globally using the command
npm install -g @angular/cli
. The Angular CLI is a command-line tool that helps you create, develop, and maintain Angular applications. - Stripe Account: Sign up for a Stripe account (https://stripe.com) and obtain your publishable and secret API keys, as you’ll need them for the integration.
Step 1: Create a new angular project
Start by creating a new Angular project using the Angular CLI:
ng new stripe-angular-app
This command will guide you through the project setup process, allowing you to configure various settings for your new Angular application, such as routing, stylesheet format, and testing framework.
Step 2: Install the stripe angular library
In your Angular project, install the Stripe Angular library using npm:
npm install @stripe/stripe-js @angular/forms
The @stripe/stripe-js
package provides the necessary Stripe functionality, while the @angular/forms
package is required for handling form-related tasks in your Angular application.
Step 3: Initialize Stripe in Your Angular Component
Open the component where you want to integrate the Stripe payment functionality (e.g., app.component.ts
) and import the necessary Stripe-related modules:
import { Component } from '@angular/core';
import { loadStripe } from '@stripe/stripe-js';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
stripePromise = loadStripe('your_stripe_publishable_key');
}
Replace 'your_stripe_publishable_key'
with your actual Stripe publishable key, which you can find in the Stripe dashboard.
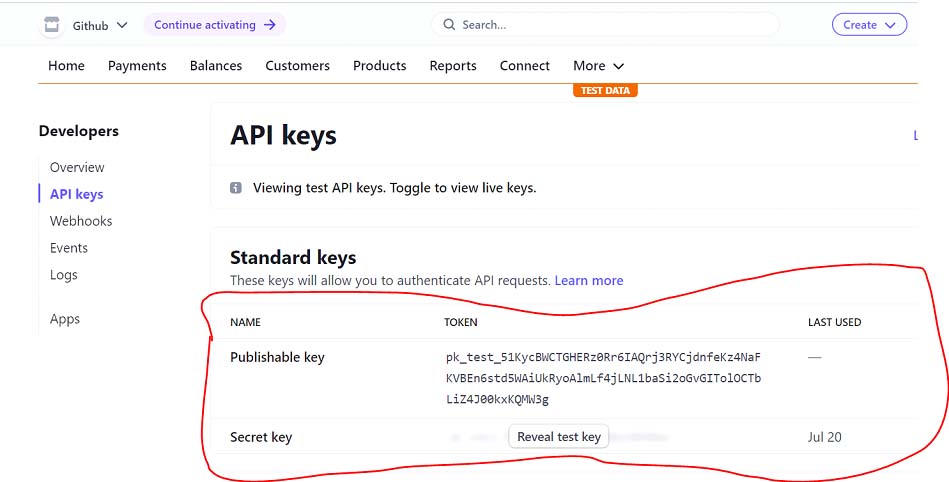
Step 4: Create a Payment Form
In your Angular component’s template (e.g., app.component.html
), add the payment form:
<form [formGroup]="paymentForm" (ngSubmit)="submitPayment()">
<div class="form-group">
<label for="card-element">Credit or Debit Card</label>
<div id="card-element"></div>
<div id="card-errors" role="alert"></div>
</div>
<button type="submit" [disabled]="paymentForm.invalid">Pay</button>
</form>
In the component’s TypeScript file (e.g., app.component.ts
), create the payment form and handle the form submission:
import { Component } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
import { StripeService, StripeCardElement, StripeCardElementOptions } from '@stripe/stripe-js';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
paymentForm: FormGroup;
card: StripeCardElement;
cardOptions: StripeCardElementOptions = {
style: {
base: {
iconColor: '#666EE8',
color: '#31325F',
fontWeight: '300',
fontFamily: '"Helvetica Neue", Helvetica, sans-serif',
fontSize: '18px',
'::placeholder': {
color: '#CFD7E0'
}
}
}
};
constructor(private formBuilder: FormBuilder, private stripeService: StripeService) {
this.paymentForm = this.formBuilder.group({
name: ['', Validators.required]
});
}
submitPayment() {
if (this.paymentForm.valid) {
this.stripeService.createToken(this.card, { name: this.paymentForm.get('name').value })
.subscribe(result => {
if (result.token) {
// Send the token to your server to process the payment
console.log(result.token.id);
} else if (result.error) {
// Display the error to the user
console.log(result.error.message);
}
});
}
}
}
In this example, we create a payment form with a credit/debit card input field using the Stripe StripeCardElement
. When the user submits the form, the component creates a Stripe token and logs it to the console (in a real-world scenario, you’d send this token to your server to process the payment).
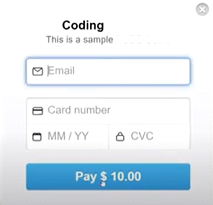
Step 5: Implement Stripe Checkout
Stripe Checkout is a hosted payment page that provides a seamless and secure checkout experience for your users. To integrate Stripe Checkout in your Angular application, you can follow these steps:
- Create a new Angular component for the Stripe Checkout functionality:
ng generate component stripe-checkout
- In the
stripe-checkout.component.ts
file, implement the Stripe Checkout integration:
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { switchMap } from 'rxjs/operators';
import { StripeService } from '@stripe/stripe-js';
@Component({
selector: 'app-stripe-checkout',
templateUrl: './stripe-checkout.component.html',
styleUrls: ['./stripe-checkout.component.css']
})
export class StripeCheckoutComponent {
constructor(private http: HttpClient, private stripeService: StripeService) {}
checkout() {
this.http.post('/create-checkout-session', {})
.pipe(
switchMap(session => {
return this.stripeService.redirectToCheckout({ sessionId: session.id });
})
)
.subscribe(result => {
if (result.error) {
alert(result.error.message);
}
});
}
}
- In the
stripe-checkout.component.html
file, add the checkout button:
<button (click)="checkout()">Proceed to Checkout</button>
- On the server-side (e.g., in a Node.js/Express.js application), create the
/create-checkout-session
endpoint:
const express = require('express');
const app = express();
const stripe = require('stripe')('your_stripe_secret_key');
app.post('/create-checkout-session', async (req, res) => {
const session = await stripe.checkout.sessions.create({
payment_method_types: ['card'],
line_items: [
{
price_data: {
currency: 'usd',
product_data: {
name: 'T-shirt',
},
unit_amount: 2000,
},
quantity: 1,
},
],
mode: 'payment',
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel',
});
res.json({ id: session.id });
});
app.listen(4242, () => console.log(`Listening on port ${4242}!`));
Replace 'your_stripe_secret_key'
with your actual Stripe secret key, which you can find in the Stripe dashboard.
By following these steps, you’ve successfully integrated Stripe Checkout into your Angular application, providing a seamless and secure checkout experience for your users.
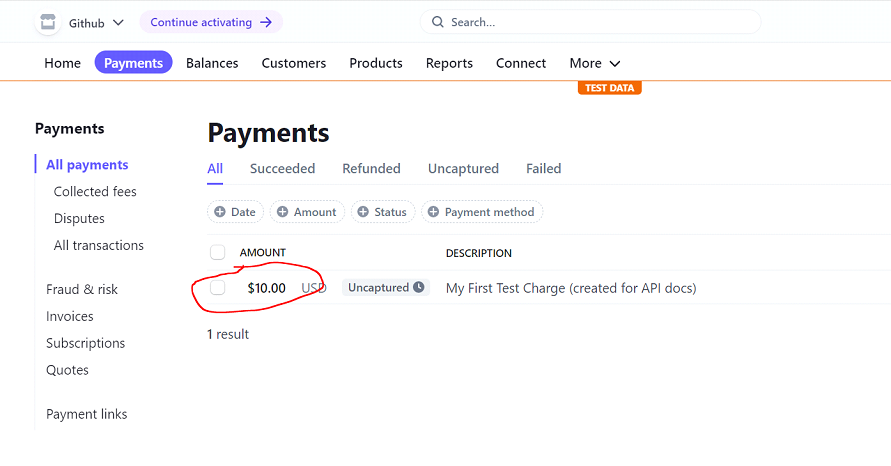
Error Handling and Customization
To enhance the user experience, you may want to add error handling and customization options to your Stripe integration.
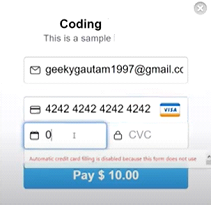
Error Handling
The Stripe Angular library provides a convenient way to handle errors that may occur during the payment process. You can listen for the change
event on the StripeCardElement
and display any errors to the user:
card.addEventListener('change', (event) => {
const displayError = document.getElementById('card-errors');
if (event.error) {
displayError.textContent = event.error.message;
} else {
displayError.textContent = '';
}
});
Customization
This library allows you to customize the appearance of the payment form elements. You can do this by passing a StripeCardElementOptions
object to the create
method:
const cardOptions: StripeCardElementOptions = {
style: {
base: {
color: '#32325d',
fontFamily: '"Helvetica Neue", Helvetica, sans-serif',
fontSmoothing: 'antialiased',
fontSize: '16px',
'::placeholder': {
color: '#aab7c4'
}
},
invalid: {
color: '#fa755a',
iconColor: '#fa755a'
}
}
};
this.card = this.elements.create('card', cardOptions);
By customizing the appearance of the payment form, you can ensure that it seamlessly integrates with the overall design of your Angular application.
Integrating Stripe with NGX-Stripe
NGX-Stripe is a popular Angular library that provides a wrapper around the Stripe Elements API, making it easier to integrate Stripe into your Angular application.
What is NGX-Stripe?
NGX-Stripe is a wrapper around the Stripe Elements. With the help of this library, you can easily add Stripe Elements to any Angular app.
Installing NGX-Stripe
- To install the latest version of NGX-Stripe, run the following command in your Angular project:
npm install ngx-stripe @stripe/stripe-js
- If you want to install a specific version for an older Angular major, use the
lts
npm tags or check the table below to pick the right version. For example, for Angular 8:
npm install ngx-stripe@v8-lts @stripe/stripe-js
Configuring NGX-Stripe
- First, import the
NgxStripeModule
into your Angular application’s main module (e.g.,app.module.ts
):
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { NgxStripeModule } from 'ngx-stripe';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
NgxStripeModule.forRoot('your_stripe_publishable_key'),
// Other modules...
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Replace 'your_stripe_publishable_key'
with your actual Stripe publishable key, which you can find in the Stripe dashboard.
Integrating Stripe Elements with NGX-Stripe
- In the component where you want to integrate the Stripe payment form, import the necessary modules and create the Stripe Elements:
import { Component } from '@angular/core';
import { NgxStripeService, StripeCardComponent, StripeElementsOptions, StripeElementOptions } from 'ngx-stripe';
@Component({
selector: 'app-stripe-payment',
templateUrl: './stripe-payment.component.html',
styleUrls: ['./stripe-payment.component.css']
})
export class StripePaymentComponent {
elementsOptions: StripeElementsOptions = {
locale: 'en'
};
cardOptions: StripeElementOptions = {
style: {
base: {
iconColor: '#666EE8',
color: '#31325F',
fontWeight: '300',
fontFamily: '"Helvetica Neue", Helvetica, sans-serif',
fontSize: '18px',
'::placeholder': {
color: '#CFD7E0'
}
}
}
};
constructor(private stripeService: NgxStripeService) {}
handlePayment() {
this.stripeService.createToken(this.cardComponent.element, {})
.subscribe((result) => {
if (result.token) {
// Handle the Stripe token
console.log(result.token.id);
} else if (result.error) {
// Handle any errors
console.log(result.error.message);
}
});
}
}
- In the corresponding template file (
stripe-payment.component.html
), add the Stripe card element:
<ngx-stripe-card
[options]="cardOptions"
[elementsOptions]="elementsOptions"
#cardComponent>
</ngx-stripe-card>
<button (click)="handlePayment()">Pay</button>
In this example, we’re using the ngx-stripe-card
component provided by the NGX-Stripe library to render the Stripe card element. We’ve also customized the appearance of the card element using the cardOptions
object.
When the user clicks the “Pay” button, the handlePayment()
method is called, which creates a Stripe token and logs it to the console (in a real-world scenario, you’d send this token to your server to process the payment).
Handling Stripe Checkout with NGX-Stripe
NGX-Stripe also provides a convenient way to integrate Stripe Checkout into your Angular application. Here’s an example:
- Create a new Angular component for the Stripe Checkout functionality:
ng generate component stripe-checkout
- In the
stripe-checkout.component.ts
file, implement the Stripe Checkout integration:
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { switchMap } from 'rxjs/operators';
import { NgxStripeService } from 'ngx-stripe';
@Component({
selector: 'app-stripe-checkout',
templateUrl: './stripe-checkout.component.html',
styleUrls: ['./stripe-checkout.component.css']
})
export class StripeCheckoutComponent {
constructor(private http: HttpClient, private stripeService: NgxStripeService) {}
checkout() {
this.http.post('/create-checkout-session', {})
.pipe(
switchMap(session => {
return this.stripeService.redirectToCheckout({ sessionId: session.id });
})
)
.subscribe(result => {
if (result.error) {
alert(result.error.message);
}
});
}
}
- In the
stripe-checkout.component.html
file, add the checkout button:
<button (click)="checkout()">Proceed to Checkout</button>
- On the server-side (e.g., in a Node.js/Express.js application), create the
/create-checkout-session
endpoint:
const express = require('express');
const app = express();
const stripe = require('stripe')('your_stripe_secret_key');
app.post('/create-checkout-session', async (req, res) => {
const session = await stripe.checkout.sessions.create({
payment_method_types: ['card'],
line_items: [
{
price_data: {
currency: 'usd',
product_data: {
name: 'T-shirt',
},
unit_amount: 2000,
},
quantity: 1,
},
],
mode: 'payment',
success_url: 'https://example.com/success',
cancel_url: 'https://example.com/cancel',
});
res.json({ id: session.id });
});
app.listen(4242, () => console.log(`Listening on port ${4242}!`));
Replace 'your_stripe_secret_key'
with your actual Stripe secret key, which you can find in the Stripe dashboard.
By following these steps, you’ve successfully integrated Stripe Checkout into your Angular application using the NGX-Stripe library, providing a seamless and secure checkout experience for your users.
Conclusion
In this comprehensive guide, we’ve covered the integration of Stripe payments with the latest version of Angular (14.x as of 2024), including the use of the NGX-Stripe library.
We have discussed about the stripe and how can we integrate stripe payment method with angular by implementing with an example of by using payment form. Further more, we have also discussed the integration of stripe with ngx stripe with an example. This will help the developer to better understand the integration of stripe with angular and integration of stripe with ngx stripe.
You can also read about the integration of stripe on demanding app and integrating apple pay with stripe. If you want to Hire Angular developer Contact.
Whether you’re building an e-commerce platform, a business website, or a complex web application, the strategies outlined in this guide will help you optimize your website’s performance, improve your search engine rankings, and outshine your competition.
If you have any further questions or need assistance with your Stripe and Angular integration, feel free to reach out to our team of experts at The Right Software. We’re always here to help you deliver the best possible solutions for your business.